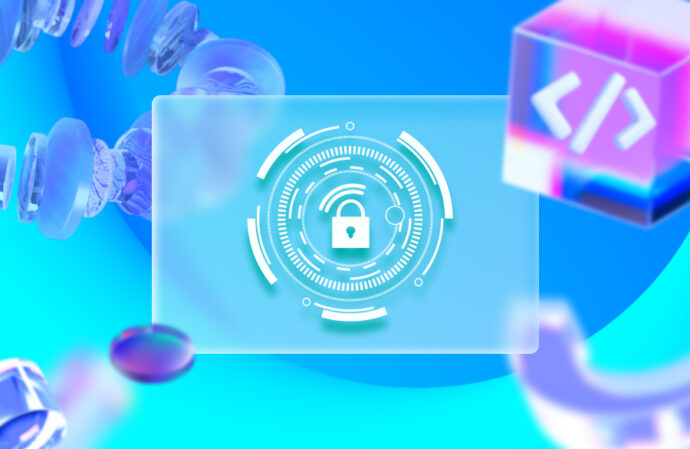
Stack-based buffer overflow attacks remain a persistent and serious security threat in the world of software development. These vulnerabilities can have far-reaching consequences, from data breaches to remote code execution. Identifying and mitigating these issues is a paramount concern for developers and security professionals. In this blog post, we will explore how CodiumAI, an innovative code analysis platform, plays a crucial role in identifying and preventing buffer overflow attacks.
Buffer overflows occur when a program writes more data into a memory buffer than it can safely hold. This excess data can overwrite adjacent memory locations, leading to unexpected and often malicious consequences. These vulnerabilities can be challenging to detect, as they often lie dormant until exploited by malicious actors.
CodiumAI is a cutting-edge tool that offers a comprehensive solution to this problem. It provides a range of features that help developers and security experts find and address buffer overflow vulnerabilities, including the ability to generate test cases, offer code explanations, and provide code suggestions to improve overall code quality and security.
In this blog, we will take a deep dive into a simple code example to illustrate how CodiumAI can identify and mitigate buffer overflow attacks. We will walk through the process of using CodiumAI to analyze the code, detect vulnerabilities, generate test cases, and understand code explanations. By the end of this blog, you will have a clearer understanding of how CodiumAI can enhance code security and make your software more robust against these potentially devastating attacks.
Note: The code we will discuss is applicable to 32-bit Linux systems only.
Let’s explore the power of CodiumAI and its role in safeguarding your software against buffer overflow vulnerabilities.
Coding example
The provided code demonstrates a buffer overflow vulnerability within the Test() function. Let’s name our code file test.c. This buffer overflow can lead to unpredictable behavior and security issues.
#include <stdio.h> #include <string.h> void Test() { char buff[5]; char buff2[3]; char buff3[4] = "abc\0"; printf("Some input: "); scanf("%s", buff); strcpy(buff2, buff); printf("buff3 is %s\n", buff3); } int main(int argc, char *argv[ ]) { Test(); return 0; }
The code above has the potential for a buffer overflow. In particular, the scanf and strcpy functions are used in a way that can lead to buffer overflows if input is not carefully controlled.
Here’s a brief analysis of the code:
- The buff array has a size of 5 characters, and the buff2 array has a size of 3 characters.
- The buff3 array is initialized with the string “abc\0” which has a size of 4 characters.
- The scanf function reads input from the user into the buff array using %s. If the input provided is longer than 5 characters, it will overflow the buff array, potentially overwriting adjacent memory.
- The strcpy function is used to copy the content of buff into buff2. If buff contains more than 3 characters, it will overflow buff2, potentially causing memory corruption.
- Finally, the program prints the content of buff3, which should be “abc” with a null terminator.
To trigger a buffer overflow, you can provide input that is longer than the size of buff when prompted for “Some input.” For example, entering a string longer than 5 characters, like “1234567,” will lead to a buffer overflow in the buff array and may result in unpredictable behavior.
Note: Intentionally triggering buffer overflows is not recommended and can have serious security and stability implications. In real-world scenarios, it’s important to avoid buffer overflows and ensure proper input validation and buffer size handling in your code.
Now, let’s examine the output produced by the code above under various input scenarios, and we will also analyze the code’s behavior.
Let’s first compile our code using the command “gcc test.c -o out”. This command compiles the C source code in the “test.c” file and produces an executable named “out.”
Let’s look at the output when the characters [1][2] are given as an input to our code:
>> ./out Some input: 12 buff3 is abc
If the provided input is [1][2] and the output of buff3 is [a][b][c], it indicates that the code did not experience a buffer overflow. In this case, [1][2] was successfully stored in the buff array, and no memory corruption occurred. The reason we are getting the output buff3 is abc is that the buff3 array is explicitly initialized with [a][b][c] and a null terminator, so it retains this value when printed.
Now let’s look at the output when the characters [1][2][3] are given as an input to our code:
>> ./out Some input: 123 buff3 is
The output buff3 is not [a][b][c] because the input [1][2][3] has a size of 4 characters [1][2][3][\0], which caused an overflow in the buff2 array when the contents were copied from buff. This caused a buffer overflow and corrupted the memory for buff3 to [\0][b][c][\0]. Therefore, we get the output buff3 is [nothing]
Now let’s look at the output when the characters [1][2][3][4] are given as an input to our code:
>> ./out Some input: 1234 buff3 is 4
In this case, the input [1][2][3][4] overflows the buff2 array as before. The [4] is the last character of our input, and it is stored in the buff3 array, overwriting its content yet again. This time, 2 characters are replaced, and now the contents of buff3 are [4][\0][c][\0]. Hence, when we print out buff3, we can see only the character [4].
Finally, when the characters [1][2][3][4][5][6] are given as an input to our code, we will get the following output:
>> ./out Segmentation fault
A “Segmentation fault” error occurs when a program attempts to access a memory location that it is not allowed to access, typically because it doesn’t have the necessary permissions or the memory is not allocated to it. In the context of our program, a segmentation fault suggests that there is a memory access violation.
In our code, the potential cause of the segmentation fault is that the input provided is too long and overflows even the buff3 array (size 4), which can lead to memory corruption and a segmentation fault.
The reason we were unable to get a segmentation fault for some inputs was because we were overwriting the memory in our own code space; once we access memory outside of our code, we get a segmentation fault error.
To troubleshoot and fix the segmentation fault, you should review your code for potential issues, including buffer overflows, and ensure that you’re not accessing memory that you’re not supposed to. Additionally, using proper memory management techniques can help avoid such errors.
Stack’s role in program execution and buffer overflow
Here’s a detailed illustration of the stack for the provided code:
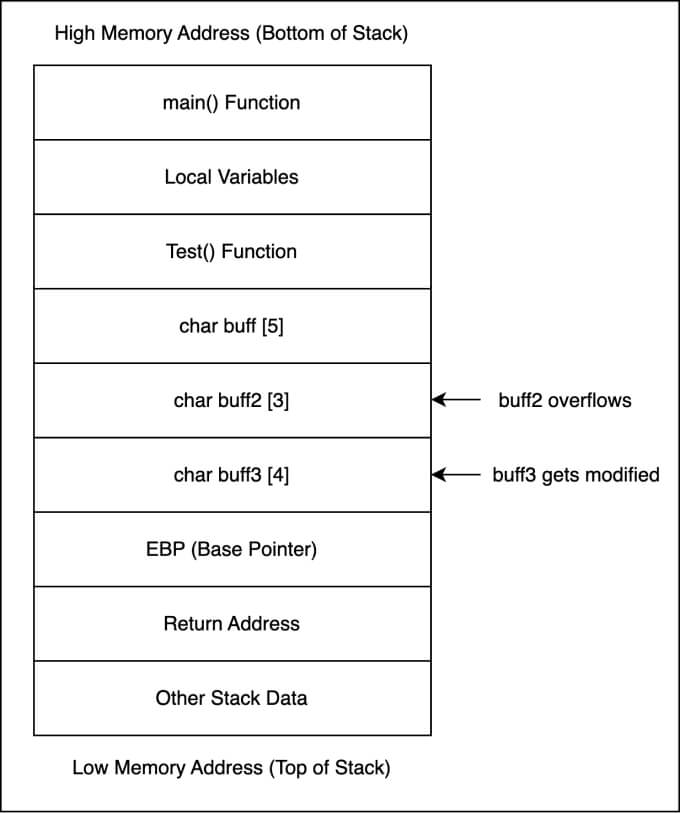
In Figure 1, the stack plays a critical role in managing the execution flow, function calls, and local variable storage. Let’s explain the stack’s role in this context:
- Function Calls and Return Addresses: When the program starts, it executes the main function. As functions are called, their return addresses (i.e., the address where execution should continue after the function completes) are pushed onto the stack.
- Stack Frames: Each function, including the main and Test functions, has its own stack frame on the stack. A stack frame is a dedicated portion of the stack that contains information about the function’s execution. This includes local variables and the function’s parameters. Local variables are specific to the function and are stored within its stack frame.
- Local Variables: Local variables, like the buffer array, are stored in the stack frame of the function to which they belong. In this case, the buffer is an array of characters allocated within the Test function’s stack frame.
- Base Pointer (EBP): The EBP (Base Pointer) is a register that points to the base of the current function’s stack frame. It helps maintain the structure of the stack frame and allows for efficient access to local variables. In the illustration, the EBP is shown relative to the buffer.
- Buffer Overflow: The buffer overflow occurs when the Test function writes data beyond the allocated space for the buffer array. This overflows into adjacent memory locations on the stack, potentially affecting the return address and other data. Buffer overflows can lead to security vulnerabilities.
- Execution Flow: The stack plays a fundamental role in managing the program’s execution flow. When a function completes, the return address on the stack is used to determine where execution should continue. In the case of a buffer overflow, if an attacker manipulates the return address, they can control the program’s execution.
In summary, the stack serves as a crucial data structure for managing function calls and local variables and controlling the program’s execution flow. The EBP and return address are essential components of the stack that help maintain the structure of function calls and ensure proper program execution. However, a buffer overflow can lead to security vulnerabilities by manipulating the stack’s content. Proper stack management and input validation are crucial to prevent such vulnerabilities.
Let’s now look at how we can use CodiumAI to prevent such a security threat.
CodiumAI: Code Explanation
Let’s now look at the code explanation generated by CodiumAI for the function overflow().
Summary
The Test() function in the given code snippet takes user input, copies it to another buffer, and prints the value of a third buffer.
Example Usage
#include <stdio.h> #include <string.h> void Test() { char buff[5]; char buff2[3]; char buff3[4] = "abc\0"; printf("Some input: "); scanf("%s", buff); strcpy(buff2, buff); printf("buff3 is %s\n", buff3); } int main(int argc, char *argv[ ]) { Test(); return 0; }
Code Analysis
Inputs
No explicit inputs are passed to the Test() function. It relies on user input through the scanf() function.
Flow
- Declare three character arrays: buff, buff2, and buff3.
- Initialize buff3 with the string abc\0.
- Print the prompt “Some input: “.
- Read user input from the console and store it in buff using scanf().
- Copy the contents of buff to buff2 using strcpy().
- Print the value of buff3 using printf().
Outputs
The output of the Test() function is the value of buff3, which is “abc”.
CodiumAI: Test case generation
Let’s now look at one of the edge cases generated by CodiumAI for the function Test():
// Function is called with input larger than 5 characters #include <stdio.h> #include <string.h> void Test() { char buff[5]; char buff2[3]; char buff3[4] = "abc\0"; printf("Some input: "); scanf("%s", buff); strcpy(buff2, buff); printf("buff3 is %s\n", buff3); } void test_input_larger_than_5_characters() { // Arrange char expected[] = "inputtoolarge"; // Act freopen("input.txt", "w", stdin); fprintf(stdin, "%s", expected); fclose(stdin); Test(); // Assert // No assertions needed as the input is larger than 5 characters }
The code contains a test function named test_input_larger_than_5_characters that is designed to test the behavior of the Test function when called with input larger than 5 characters. Here’s an explanation of how the test function works:
- In the “Arrange” section, a character array expected is defined, representing the input that is larger than 5 characters.
- In the “Act” section, the standard input (stdin) is redirected to a file named “input.txt” to simulate user input. The fprintf function is used to write the expected input to stdin. This input is larger than 5 characters.
- The Test function is then called, and it reads the input from stdin.
- In the “Assert” section, there are no specific assertions provided in the code. However, the comment states that no assertions are needed because the input is intentionally larger than 5 characters.
This test is meant to observe the behavior of the Test function when given input that exceeds the capacity of the buff array (which has a size of 5 characters) to see how it handles such input. The test case above is going to fail because the input provided is larger than 5 characters. To fix this issue, we will now look at a suggestion provided by CodiumAI.
CodiumAI: Code Suggestion
This code recommendation pertains to the best practices for addressing an important vulnerability. Let’s now finally look at one of the code suggestions provided by CodiumAI:
Suggestion
Replace scanf with fgets to prevent buffer overflow.
Why
Buffer overflow is a common vulnerability that can lead to security issues such as arbitrary code execution or crashing the program. Using fgets instead of scanf allows specifying the maximum number of characters to read, preventing buffer overflow.
Base Code
// line number: 4 void Test() { char buff[5]; char buff2[3]; char buff3[4] = "abc\0"; printf("Some input: "); scanf("%s", buff); strcpy(buff2, buff); printf("buff3 is %s\n", buff3); }
Suggested Code
void Test() { char buff[5]; char buff2[3]; char buff3[4] = "abc\0"; printf("Some input: "); fgets(buff, sizeof(buff), stdin); strcpy(buff2, buff); printf("buff3 is %s\n", buff3); }
Now let’s look at the output when the characters “123” are given as input to our updated code:
>> ./out Some input: 123 buff3 is abc
In this context, it’s evident that the proposed code effectively mitigated the buffer overflow vulnerability.
Conclusion
In conclusion, buffer overflow attacks pose an enduring and serious threat in the world of software development, making them a top priority for both developers and security experts. The potential consequences, ranging from data breaches to remote code execution, underscore the urgency of identifying and mitigating these vulnerabilities effectively.
CodiumAI, an advanced code analysis platform, plays a pivotal role in addressing this challenge. Its comprehensive set of features empowers developers and security professionals to not only detect but also proactively prevent buffer overflow vulnerabilities. With capabilities that include test case generation, code explanations, and quality-enhancing suggestions, CodiumAI provides a robust defense against these critical security risks.
Our exploration of a simple code example has revealed how CodiumAI excels at identifying and mitigating stack-based buffer overflow attacks. This tool goes beyond mere detection; it equips you with the means to reinforce your code’s security, enhancing its resistance to potential threats.
By harnessing the power of CodiumAI, you can fortify your software against buffer overflow vulnerabilities and make your applications more robust and secure.