Problem Formulation
π‘ Question: How do you convert or flatten a list of strings to a single string with and without a separator?
Here are five examples:
- No Separator
- Input:
["Hello", "World"]
- Output:
"HelloWorld"
- Input:
- Space Separator
- Input:
["Hello", "World"]
- Output:
"Hello World"
- Input:
- Comma Separator
- Input:
["apple", "banana", "cherry"]
- Output:
"apple,banana,cherry"
- Input:
- Newline Separator
- Input:
["line1", "line2", "line3"]
- Output:
"line1\nline2\nline3"
- Input:
- Hyphen Separator
- Input:
["1", "2", "3", "4", "5"]
- Output:
"1-2-3-4-5"
- Input:
In each case, the .join()
method can be used in Python with the specified separator to achieve the desired output. π
Method 1: Using the join() Method
The join()
method is a straightforward and efficient way to concatenate a list of strings. It allows you to specify a separator, which can be any string, including an empty string, to join the elements in the list.
fruits = ["apple", "banana", "cherry"] result = " ".join(fruits) print(result)
This code concatenates the strings in the fruits
list, separated by a space. The output will be 'apple banana cherry'
.
π Python Converting List of Strings to * [Ultimate Guide]
Method 2: Using a For Loop
Iterating over the list with a for
loop and concatenating each element to a new string. This method offers more control over the concatenation process.
fruits = ["apple", "banana", "cherry"] result = "" for fruit in fruits: result += fruit + " " print(result.strip())
The code iterates through each string in fruits
, adding them to result
with a space. The strip()
function is used to remove the trailing space.
π How to Iterate Over a List?
Method 3: Using List Comprehension
This method involves using list comprehension to create a new list of strings and then joining them. Itβs a more concise version of the for
loop approach but really only a trivial variation of the first method. Not recommended!
fruits = ["apple", "banana", "cherry"] result = " ".join([fruit for fruit in fruits]) print(result)
The list comprehension [fruit for fruit in fruits]
creates a new list identical to fruits
, and join()
concatenates them with a space.
π Python List Comprehension
Method 4: Using the map() Function
The map()
function applies a given function to each item of an iterable (like a list) and returns a list of the results. When combined with join()
, it can be used for string concatenation.
fruits = ["apple", "banana", "cherry"] result = " ".join(map(str, fruits)) print(result)
The map(str, fruits)
converts each element in fruits
to a string (if not already), and join()
concatenates them.
Method 5: Using reduce() from functools
The reduce()
function, which is part of the functools
module, applies a function cumulatively to the items of a list, from left to right, so as to reduce the list to a single value.
from functools import reduce fruits = ["apple", "banana", "cherry"] result = reduce(lambda a, b: a + " " + b, fruits) print(result)
The reduce()
function applies the lambda function cumulatively to the elements of fruits
, concatenating them with a space.
Comparison and Tips
- Efficiency:
join()
is generally the most efficient method, especially for large lists. - Flexibility: Loops and list comprehension offer more control over the concatenation process.
- Simplicity:
join()
andmap()
are more readable and concise. - Use Case: Use
join()
for simple concatenations; loops or list comprehension for more complex conditions;map()
for applying a function to elements; andreduce()
for cumulative concatenations.
Related Video
π Whatβs the most Pythonic way to join a list of objects?
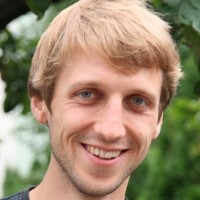
While working as a researcher in distributed systems, Dr. Christian Mayer found his love for teaching computer science students.
To help students reach higher levels of Python success, he founded the programming education website Finxter.com that has taught exponential skills to millions of coders worldwide. Heβs the author of the best-selling programming books Python One-Liners (NoStarch 2020), The Art of Clean Code (NoStarch 2022), and The Book of Dash (NoStarch 2022). Chris also coauthored the Coffee Break Python series of self-published books. Heβs a computer science enthusiast, freelancer, and owner of one of the top 10 largest Python blogs worldwide.
His passions are writing, reading, and coding. But his greatest passion is to serve aspiring coders through Finxter and help them to boost their skills. You can join his free email academy here.