Introduction
Imagine introducing your grandmother to the marvels of artificial intelligence by letting her interact with ChatGPT, witnessing her excitement as the AI engages in a seamless conversation. This article explores how you can create your own intelligent AI agents using LangChain, a powerful Python library that simplifies the development process.
By leveraging LangChain, even those with minimal technical expertise can build sophisticated AI applications tailored to specific needs. We’ll guide you through setting up an AI agent capable of web scraping and content summarizing, showcasing the potential of LangChain to transform how you approach various tasks. Whether you’re a novice or an industry expert, LangChain equips you with the tools to develop dynamic and context-aware AI solutions.
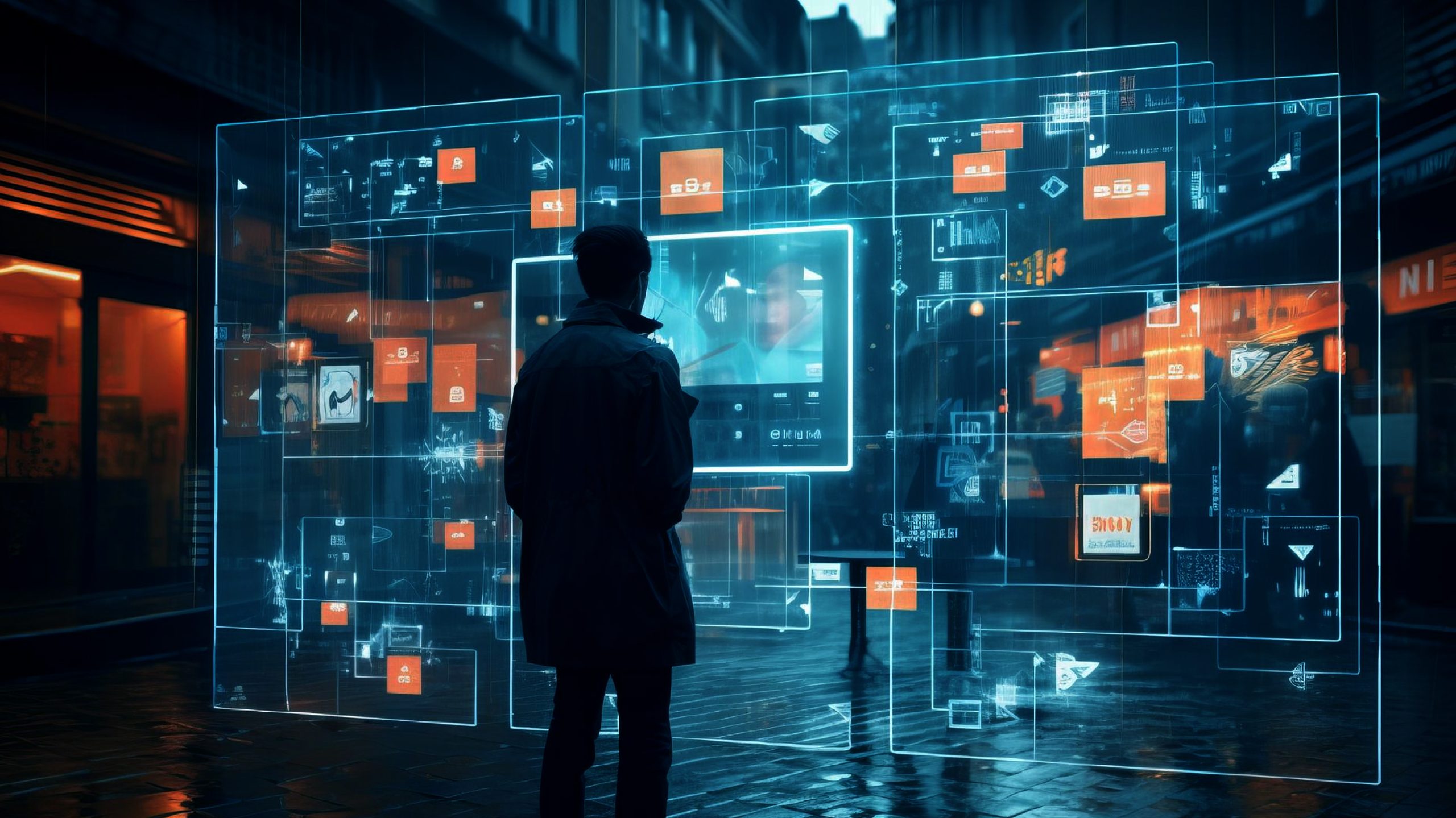
Overview
- Understand the core features and benefits of using LangChain for AI agent development.
- Learn how to set up and configure LangChain in a Python environment.
- Gain practical experience in building AI agents for tasks like web scraping and content summarization.
- Discover the differences between traditional chatbots and LangChain agents.
- Explore how to customize and extend LangChain to suit specific application needs.
What is LangChain?
LangChain streamlines the development of intelligent AI agents with its innovative open-source library. In the rapidly evolving field of artificial intelligence (AI), the ability to create agents that can engage in natural and contextually aware dialogues with users has become increasingly valuable. LangChain stands out by providing a robust framework that integrates seamlessly with various language models, making it an ideal choice for developers aiming to build sophisticated AI agents.
Definition and Context
LangChain addresses the need for more advanced and versatile AI agents. Traditional chatbots, while useful, often fall short in maintaining context and understanding nuanced interactions. LangChain addresses these limitations by leveraging state-of-the-art language models, such as GPT-3, to enhance the conversational capabilities of the agents it powers.
The library emerged from the recognition that while powerful language models exist, integrating them into practical applications can be challenging. LangChain abstracts much of this complexity, offering a user-friendly interface that simplifies the process of building, training, and deploying AI agents.
Key Features of LangChain
LangChain offers a suite of features designed to facilitate the development of robust AI agents. One of its primary strengths is its modular architecture, which allows developers to mix and match components according to their needs. This flexibility ensures that LangChain can be tailored to a wide variety of use cases, from customer service bots to virtual personal assistants.
- Integration with Advanced Language Models: LangChain supports the integration of cutting-edge language models like GPT-3, enabling agents to generate more natural and contextually appropriate responses. This capability is crucial for creating engaging user interactions that mimic human-like conversation.
- Context Management: One of the standout features of LangChain is its ability to maintain context throughout a conversation.
- Customizability and Extensibility: LangChain is designed to be highly customizable. Developers can extend its functionality by integrating additional APIs and data sources, tailoring the behavior of their agents to meet specific requirements.
- Ease of Use: Despite its powerful capabilities, LangChain remains user-friendly by design.
Fundamentals of LangChain Agents
In the LangChain documentation, we can read: “The core idea of agents is to use a language model to choose a sequence of actions to take. A sequence of actions is hardcoded (in code) in chains. In agents, a language model is used as a reasoning engine to determine which actions to take and in which order.”
An agent, in the context of AI, refers to a more advanced and autonomous system capable of performing a broader range of tasks. Agents are designed to understand, interpret, and respond to user inputs more flexibly and intelligently compared to chatbots. In other words, the agents allow you to simply do the task on your behalf.
After that, you can tell me what is the difference with a classic chatbot. Unlike agents, a chatbot is a computer program designed to simulate conversation with human users, especially over the internet. The big difference is in the use of LLM and deep learning algorithms to generate responses dynamically. They are not limited to scripted interactions and can adapt their responses based on the context and nuances of the conversation. Unlike traditional chatbots that often struggle with context retention, LangChain can remember previous interactions, making dialogues more coherent and relevant over extended interactions.
Hands-On Code Example: Building an AI Agent
To demonstrate the use of an agent with web scraping and the fundus library, we can create a Python script that uses LangChain to build an agent that scrapes articles from the web and summarizes them.
You’ll need a Python environment configured with the necessary libraries to get started. Here’s how to install LangChain :
pip install langchain fundus
Imports
from langchain.agents import tool
from langchain_openai import ChatOpenAI
from fundus import PublisherCollection, Crawler, Requires
from langchain_core.prompts import ChatPromptTemplate, MessagesPlaceholder
Initializing the LLM
llm = ChatOpenAI(model=”gpt-3.5-turbo”, temperature=0)
ChatOpenAI: Initializes an instance of the GPT-3.5 model with a temperature setting of 0, which makes the model’s responses more deterministic.
In this section, we extract an article from a USA news publisher with fundus library.
@tool
def extract_article(max_article: int):
"""Returns a news article article from USA."""
crawler = Crawler(PublisherCollection.us)
article_extracted = [article.body.text() for article in crawler.crawl(max_articles=max_article)][0]
return str(article_extracted)
- @tool: Decorator that defines the function as a tool for the agent.
- Crawler(PublisherCollection.us): Initializes a crawler for the US publisher collection.
- crawler.crawl(max_articles=max_article): Crawls the website for the specified number of articles.
- article.body.text(): Extracts the text of the article body.
- return str(article_extracted): Returns the extracted article text as a string.
Example Article Text
The article is:
The families of civilians killed by the U.S. in Somalia share their ideas of justice in a new report. The Pentagon has no response. The American military has been carrying out a continuous military campaign in Somalia since the 2000s, launching nearly 300 drone strikes and commando raids over the past 17 years. In one April 2018 air attack, American troops killed three, and possibly five, civilians with a pair of missiles. A U.S. military investigation reported that the second missile killed a woman and child, but the report concluded their identities might remain unknown.
Last year, my investigation for The Intercept exposed the details of this disastrous attack. The second missile killed 22-year-old Luul Dahir Mohamed and her 4-year-old daughter, Mariam Shilow Muse, after they survived the initial strike.
This is an example of the article text that might be extracted by the extract_article tool.
Listing Tool
tools = [extract_article]
Prompt Template
prompt = ChatPromptTemplate.from_messages(
[
("system", "You are very powerful assistant, but don't know current events"),
("user", "{input}"),
MessagesPlaceholder(variable_name="agent_scratchpad"),
]
)
- ChatPromptTemplate.from_messages: Creates a prompt template for the agent.
- MessagesPlaceholder: Placeholder for the agent’s scratchpad (intermediate steps).
Binding Tools to LLM
This code binds the specified tools to the language model (`llm`) to enhance its functionality.
llm_with_tools = llm.bind_tools(tools)
Setting Up the Agent
This code sets up an agent by combining input handling, prompt formatting, LLM tool integration, and output parsing using the `format_to_openai_tool_messages` and `OpenAIToolsAgentOutputParser` functions.
from langchain.agents.format_scratchpad.openai_tools import format_to_openai_tool_messages
from langchain.agents.output_parsers.openai_tools import OpenAIToolsAgentOutputParser
agent = (
{
"input": lambda x: x["input"],
"agent_scratchpad": lambda x: format_to_openai_tool_messages(x["intermediate_steps"]),
}
| prompt
| llm_with_tools
| OpenAIToolsAgentOutputParser()
)
- format_to_openai_tool_messages: Formats intermediate steps for the agent.
- OpenAIToolsAgentOutputParser: Parses the output from the OpenAI tools.
- agent: Combines all components (input handling, prompt, LLM with tools, and output parser) into a single agent.
Executing the Agent
This code initializes an `AgentExecutor` to run the agent with the specified tools and enables detailed logging by setting `verbose=True`.
from langchain.agents import AgentExecutor
agent_executor = AgentExecutor(agent=agent, tools=tools, verbose=True)
- AgentExecutor: Executes the agent with the specified tools and settings.
- verbose=True: Enables detailed logging of the agent’s actions.
Running and Testing the Agent
result = list(agent_executor.stream({"input": "What is about this article?"}))
agent_executor.stream({“input”: “What is about this article in few words ?”}): Runs the agent with the input question, streaming the response.
Output:
result[2]['output']
The article discusses the lack of accountability and justice for civilian victims of U.S. drone strikes in Somalia. It highlights the families’ desire for acknowledgment, apologies, and financial compensation, which the U.S. government has not provided despite years of military operations and civilian casualties.
Summary of Code
This script sets up an AI-powered agent using LangChain and integrates web scraping capabilities with the fundus library. The agent scrapes articles, processes data, and answers queries based on the extracted content. This example shows how to create a versatile AI agent for real-world tasks such as data extraction and analysis.
Conclusion
This article covers how to use LangChain to develop smart AI agents for tasks like content summarizing and web scraping. First, the GPT-3.5 model is initialized, and tools for retrieving articles from news sources are defined. After that, the tutorial walks through designing an agent to answer user inquiries, attaching tools to the language model, and creating a prompt template.
Frequently Asked Questions
A. LangChain is a Python library that streamlines AI agent development with standardized interfaces, prompt management, and tool integration.
A. LangChain AI agents use language models to perform actions based on user input, enabling more dynamic and context-aware interactions.
A. LangChain agents use language models for natural, context-aware responses, unlike traditional chatbots with scripted interactions.